sync_time_ntp_totalxsoftware 0.0.3
sync_time_ntp_totalxsoftware: ^0.0.3 copied to clipboard
A Flutter utility library for validating device time synchronization with an NTP server. It helps detect whether the device's date and time are accurate, fetches NTP network time, and offers settings [...]
sync_time_ntp_totalxsoftware #
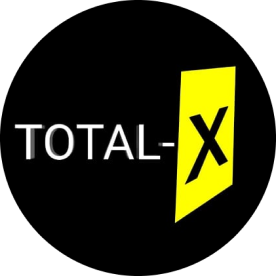
Developed by Totalx Software
A Flutter package for checking and synchronizing the device time with NTP (Network Time Protocol) server time. This package ensures that the device's time is accurate within a configurable tolerance and provides user-friendly dialogs to prompt corrections when discrepancies are detected.
Features #
- Fetch accurate time from an NTP server.
- Validate device time against NTP time with configurable tolerance.
- Display dialogs for time issues and guide users to date/time settings.
- Supports both Android and iOS platforms for settings navigation.
Installation #
Add the following dependencies to your project's pubspec.yaml
file:
dependencies:
sync_time_ntp_totalxsoftware: ^0.0.3
Run the following command:
flutter pub get
Usage #
1. Validate Device Time #
Check if the device time is synchronized with the NTP server:
import 'package:sync_time_ntp_totalxsoftware/sync_time_ntp_totalxsoftware.dart';
void validateTime() async {
bool isTimeSynced = await NtpTimeSyncChecker.validateDeviceTime(
toleranceInSeconds: 5,
);
if (isTimeSynced) {
print("Device time is synchronized.");
} else {
print("Device time is NOT synchronized.");
}
}
2. Fetch NTP Network Time #
Fetch the current accurate time from an NTP server:
import 'package:sync_time_ntp_totalxsoftware/sync_time_ntp_totalxsoftware.dart';
void fetchNTPTime() async {
DateTime? networkTime = await NtpTimeSyncChecker.getNetworkTime();
if (networkTime != null) {
print("Network Time: \$networkTime");
} else {
print("Failed to fetch NTP time.");
}
}
3. Show Time Sync Issue Dialog #
Display a dialog when the device's time is not synced, prompting the user to correct it:
import 'package:flutter/material.dart';
import 'package:sync_time_ntp_totalxsoftware/sync_time_ntp_totalxsoftware.dart';
// Show a dialog if time is not synchronized
NtpTimeSyncChecker.showTimeSyncIssueDialog(
context: context,
onRetry: () {
Navigator.pop(context);
_validateTime();
},
);
4. Complete Example #
Here is a full example showcasing the package usage:
import 'package:flutter/material.dart';
import 'package:sync_time_ntp_totalxsoftware/sync_time_ntp_totalxsoftware.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'NTP Time Sync Example',
home: const HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
String _status = "Not Checked";
String _networkTime = "-";
Future<void> _validateTime() async {
bool isSynced = await NtpTimeSyncChecker.validateDeviceTime(
toleranceInSeconds: 5,
);
setState(() {
_status = isSynced ? "Time is synchronized" : "Time is NOT synchronized!";
});
if (!isSynced) {
NtpTimeSyncChecker.showTimeIssueDialog(
context: context,
onRetry: _validateTime,
);
}
}
Future<void> _fetchNetworkTime() async {
DateTime? time = await NtpTimeSyncChecker.getNetworkTime();
setState(() {
_networkTime = time?.toString() ?? "Failed to fetch time";
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('NTP Time Sync Example')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text('Network Time: $_networkTime'),
ElevatedButton(
onPressed: _fetchNetworkTime,
child: const Text('Fetch NTP Time'),
),
const SizedBox(height: 20),
Text('Status: $_status'),
ElevatedButton(
onPressed: _validateTime,
child: const Text('Validate Device Time'),
),
],
),
),
);
}
}
API Reference #
validateTime({int toleranceInSeconds = 5}) → Future<bool>
#
- Description: Validates if the device's time is within a specified tolerance compared to the NTP server.
- Parameters:
toleranceInSeconds
: The acceptable difference in seconds (default:5
seconds).
- Returns:
true
if synchronized, otherwisefalse
.
getNetworkTime() → Future<DateTime?>
#
- Description: Fetches the current accurate time from an NTP server.
- Returns: A
DateTime
object with the network time ornull
on failure.
showTimeIssueDialog({required BuildContext context, required void Function() onRetry})
#
- Description: Displays a dialog prompting the user to fix time synchronization issues.
- Parameters:
context
: The widget context for displaying the dialog.onRetry
: A callback for retrying the time validation.
Supported Platforms #
- Android: Opens the date/time settings.
- iOS: Opens the date/time settings.
Explore more about TotalX at www.totalx.in - Your trusted software development company! #
🌐 Connect with Totalx Software #
Join the vibrant Flutter Firebase Kerala community for updates, discussions, and support: